This release adds support for three new features:
- Choose which bands to use for RGB channels when tile serving
- Generate thumbnails with the same styling parameters as tile serving
- Use any Matplotlib colormap as a `palette` choice
Example
There is a new example in the README to demonstrate RGB channel selection:
py
from localtileserver import get_leaflet_tile_layer, TileClient
from ipyleaflet import Map, ScaleControl, FullScreenControl, SplitMapControl
First, create a tile server from local raster file
tile_client = TileClient('landsat.tif')
Create 2 tile layers from same raster viewing different bands
l = get_leaflet_tile_layer(tile_client, band=[7, 5, 4])
r = get_leaflet_tile_layer(tile_client, band=[5, 3, 2])
Make the ipyleaflet map
m = Map(center=tile_client.center(), zoom=12)
control = SplitMapControl(left_layer=l, right_layer=r)
m.add_control(control)
m.add_control(ScaleControl(position='bottomleft'))
m.add_control(FullScreenControl())
m
<img width="841" alt="ipyleaflet-multi-bands" src="https://user-images.githubusercontent.com/22067021/144772211-53c1d854-ecdd-4f19-9594-4843303e2b04.png">
Thumbnails
and you can also generate styled thumbnails with
py
tile_client.thumbnail(band=[5, 3, 2], output_path='thumbnail_styled.png')
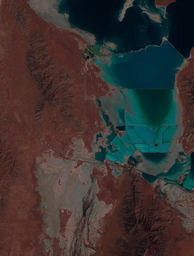
as opposed to the default channels:
py
tile_client.thumbnail(output_path='thumbnail_default.png')
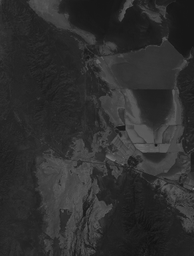
Matplotlib Colormaps
and you can plot any single band with a matplotlib colormap by:
py
l = get_leaflet_tile_layer(tile_client, band=7, palette='rainbow')
m = Map(center=tile_client.center(), zoom=10)
m.add_layer(l)
m
<img width="1159" alt="Screen Shot 2021-12-05 at 6 15 32 PM 1" src="https://user-images.githubusercontent.com/22067021/144772441-a4c656f9-76df-4900-bbc4-9a0939b0705d.png">